SalesforceのJSONとは?概要やサンプルプログラムをご紹介
IT・技術関連
更新日:2024.09.05

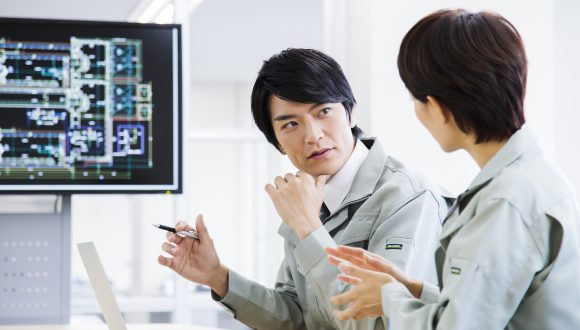
JSONとは?
JSONとは「JavaScript Object Notation」の略で、JavaScriptのオブジェクト表記を使ったデータのフォーマットのことを指します。
JSONは、Python、PHP、JavaScript、C++、Javaなど様々な言語でサポートされているので、JSONを使用すれば異なる言語間でのデータ受け渡しが簡単に行えます。
例えば、クライアント側のJavaScriptと、サーバ側のPythonのデータ受け渡しなどで使用されています。
SalesforceのJSONサポート
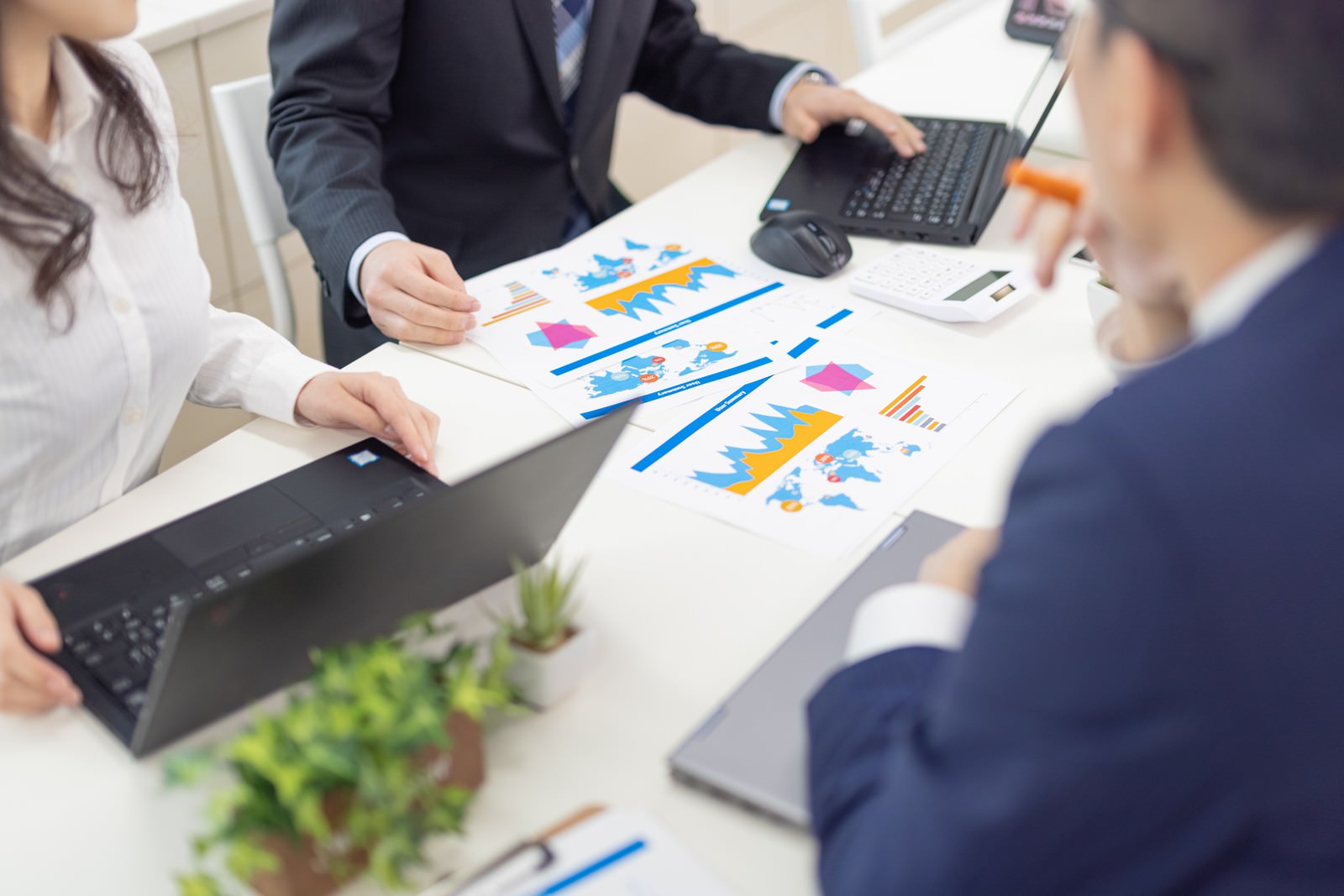
System.JSONクラス
SalesforceのSystem.JSONクラスは、ApexオブジェクトをJSON形式で逐次化したり、JSONコンテンツを並列化することが可能です。 System.JSONクラスのメソッドを使用すれば、ApexオブジェクトのJSON逐次化と並列化の往復処理を行うことができます。System.JSONクラスのサンプル
System.JSONクラスのメソッドを使用して、JSONの逐次化と並列化の往復処理を行うサンプルプログラムです。 サンプルプログラムでは、SalesforceのInvoiceStatementリストを作成し逐次化しています。逐次化されたJSON文字列を使ってリストを並列化し、元のリストと同じ請求書が新しいリストに含まれることを検証しています。
public class JSONRoundTripSample {
public class InvoiceStatement {
Long invoiceNumber;
Datetime statementDate;
Decimal totalPrice;
public InvoiceStatement(Long i, Datetime dt, Decimal price)
{
invoiceNumber = i;
statementDate = dt;
totalPrice = price;
}
}
public static void SerializeRoundtrip() {
Datetime dt = Datetime.now();
// Create a few invoices.
InvoiceStatement inv1 = new InvoiceStatement(1,Datetime.valueOf(dt),1000);
InvoiceStatement inv2 = new InvoiceStatement(2,Datetime.valueOf(dt),500);
// Add the invoices to a list.
List invoices = new List();
invoices.add(inv1);
invoices.add(inv2);
// Serialize the list of InvoiceStatement objects.
String JSONString = JSON.serialize(invoices);
System.debug('Serialized list of invoices into JSON format: ' + JSONString);
// Deserialize the list of invoices from the JSON string.
List deserializedInvoices =
(List)JSON.deserialize(JSONString, List.class);
System.assertEquals(invoices.size(), deserializedInvoices.size());
Integer i=0;
for (InvoiceStatement deserializedInvoice :deserializedInvoices) {
system.debug('Deserialized:' + deserializedInvoice.invoiceNumber + ','
+ deserializedInvoice.statementDate.formatGmt('MM/dd/yyyy HH:mm:ss.SSS')
+ ', ' + deserializedInvoice.totalPrice);
system.debug('Original:' + invoices[i].invoiceNumber + ','
+ invoices[i].statementDate.formatGmt('MM/dd/yyyy HH:mm:ss.SSS')
+ ', ' + invoices[i].totalPrice);
i++;
}
}
}
System.JSONGeneratorクラス
SalesforceのSystem.JSONGeneratorクラスは、標準JSON符号化方式を使ってオブジェクトをJSONコンテンツに逐次化するなどが可能です。 System.JSONGeneratorクラスのメソッドを使用すれば、標準JSONで符号化されたコンテンツを生成することができます。System.JSONGeneratorクラスのサンプル
System.JSONGeneratorクラスのメソッドを使用して、標準JSON符号化方式のコンテンツを生成するサンプルプログラムです。 サンプルプログラムでは、Salesforce上で見栄えの良い印刷向けのJSON文字列を生成しています。
public class JSONGeneratorSample{
public class A {
String str;
public A(String s) { str = s; }
}
static void generateJSONContent() {
// Create a JSONGenerator object.
// Pass true to the constructor for pretty print formatting.
JSONGenerator gen = JSON.createGenerator(true);
// Create a list of integers to write to the JSON string.
List intlist = new List();
intlist.add(1);
intlist.add(2);
intlist.add(3);
// Create an object to write to the JSON string.
A x = new A('X');
// Write data to the JSON string.
gen.writeStartObject();
gen.writeNumberField('abc', 1.21);
gen.writeStringField('def', 'xyz');
gen.writeFieldName('ghi');
gen.writeStartObject();
gen.writeObjectField('aaa', intlist);
gen.writeEndObject();
gen.writeFieldName('Object A');
gen.writeObject(x);
gen.writeEndObject();
// Get the JSON string.
String pretty = gen.getAsString();
System.assertEquals('{\n' +
' "abc" : 1.21,\n' +
' "def" : "xyz",\n' +
' "ghi" : {\n' +
' "aaa" : [ 1, 2, 3 ]\n' +
' },\n' +
' "Object A" : {\n' +
' "str" : "X"\n' +
' }\n' +
'}', pretty);
}
}
System.JSONParserクラス
SalesforceのSystem.JSONParserクラスは、JSON符号化されたコンテンツを変換して解析することが可能です。 System.JSONParserクラスのメソッドを使用すれば、例えばWebサービスのコールアウトのJSON符号化方式の応答などを解析することができます。System.JSONParserクラスのサンプル
SalesforceのJSONParserクラスのメソッドを使用して、外部サービスから受け取ったJSON形式の応答を解析するサンプルプログラムです。 サンプルプログラムでは、Salesforce上においてJSON形式で応答するWebサービスのコールアウトを行い、受け取った応答を解析して価格の統計を計算しています。
public class JSONParserUtil {
@future(callout=true)
public static void parseJSONResponse() {
Http httpProtocol = new Http();
// Create HTTP request to send.
HttpRequest request = new HttpRequest();
// Set the endpoint URL.
String endpoint = 'https://docsample.herokuapp.com/jsonSample';
request.setEndPoint(endpoint);
// Set the HTTP verb to GET.
request.setMethod('GET');
// Send the HTTP request and get the response.
// The response is in JSON format.
HttpResponse response = httpProtocol.send(request);
System.debug(response.getBody());
/* The JSON response returned is the following:
String s = '{"invoiceList":[' +
'{"totalPrice":5.5,"statementDate":"2011-10-04T16:58:54.858Z","lineItems":[' +
'{"UnitPrice":1.0,"Quantity":5.0,"ProductName":"Pencil"},' +
'{"UnitPrice":0.5,"Quantity":1.0,"ProductName":"Eraser"}],' +
'"invoiceNumber":1},' +
'{"totalPrice":11.5,"statementDate":"2011-10-04T16:58:54.858Z","lineItems":[' +
'{"UnitPrice":6.0,"Quantity":1.0,"ProductName":"Notebook"},' +
'{"UnitPrice":2.5,"Quantity":1.0,"ProductName":"Ruler"},' +
'{"UnitPrice":1.5,"Quantity":2.0,"ProductName":"Pen"}],"invoiceNumber":2}' +
']}';
*/
// Parse JSON response to get all the totalPrice field values.
JSONParser parser = JSON.createParser(response.getBody());
Double grandTotal = 0.0;
while (parser.nextToken() != null) {
if ((parser.getCurrentToken() == JSONToken.FIELD_NAME) &&
(parser.getText() == 'totalPrice')) {
// Get the value.
parser.nextToken();
// Compute the grand total price for all invoices.
grandTotal += parser.getDoubleValue();
}
}
system.debug('Grand total=' + grandTotal);
}
}
SalesforceでJSONを使いこなそう!
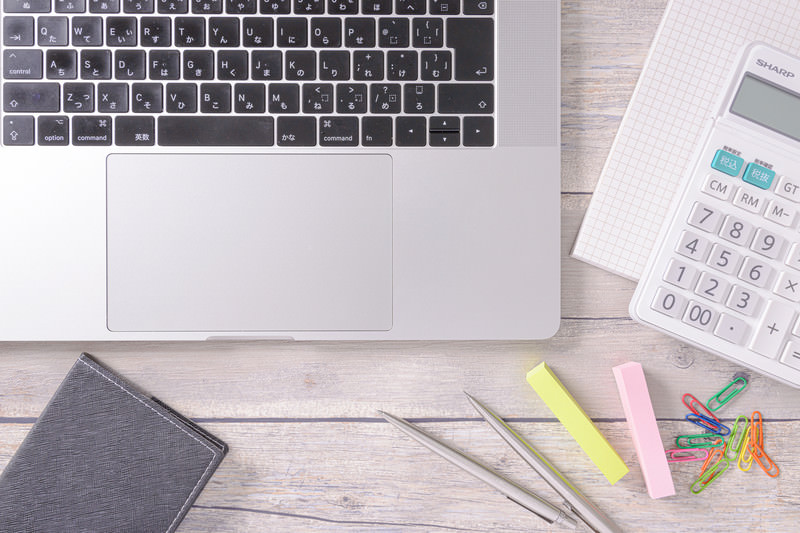
この記事の監修者・著者

- AWSパートナー/Salesforce認定コンサルティングパートナー 認定企業
-
ITエンジニア派遣サービス事業を行っています。AWSやSalesforceなど専門領域に特化したITエンジニアが4,715名在籍し、常時100名以上のITエンジニアの即日派遣が可能です。
・2021年:AWS Japan Certification Award 2020 ライジングスター of the Year 受賞
・2022年3月:人材サービス型 AWSパートナー認定
・AWS認定資格保有者数1,154名(2024年6月現在)
・Salesforce認定コンサルティングパートナー
・Salesforce認定資格者276名在籍(2024年5月現在)
・LPIC+CCNA 認定資格者:472 名(2024年6月時点)
最新の投稿
- 2024-12-27営業インタビュー情報共有の活性化の中心に。SP企画部の新たな取り組み
- 2024-07-01営業インタビュー最短で当日にご提案可能。 OPE営業の対応が早い3つの理由
- 2024-07-01営業インタビュー研修見学ツアーが高評価!「お客様のOPEに対する期待を高め、継続に貢献できればと思います。」
- 2024-07-01営業インタビュー信頼関係を構築し、エンジニアの長期就業へ
ITエンジニアの派遣を利用したい企業様へ
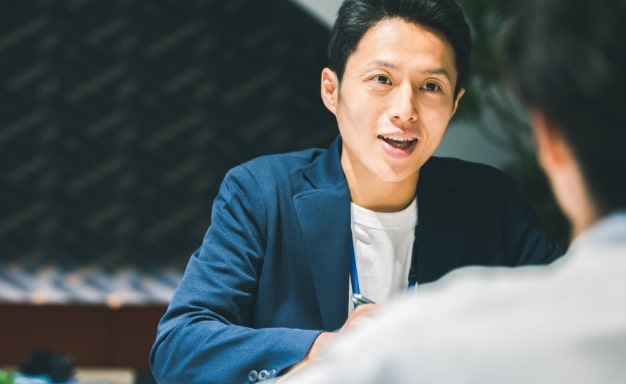
- 求人・転職サイトや自社採用サイトを使っているが、自社に合ったITエンジニアが応募してこない…
- すぐに採用したいが、応募がぜんぜん集まらない
こんな悩みをお持ちの採用・人事担当者の方は、
オープンアップITエンジニアをご検討ください!
オープンアップITエンジニアをご検討ください!
当社のITエンジニア派遣サービスは
- 派遣スピードが速い!(最短即日)
- 4,500名のエンジニアから貴社にマッチした人材を派遣
- 正社員雇用も可能
こんな特長があり、貴社の事業やプロジェクトに合った最適なITエンジニアを派遣可能です。
まずは下記ボタンから無料でご相談ください。